Jquery实时改变网页设计(背景色)
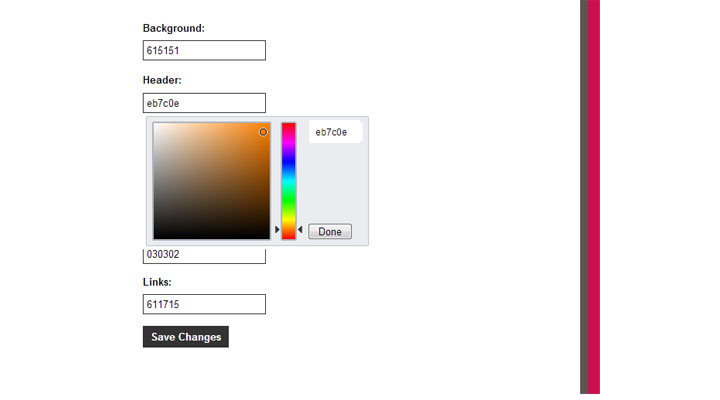
PHP Code
- <?php
- include('conn.php');
- if($_SERVER["REQUEST_METHOD"] == "POST")
- {
- $background=$_POST['background'];
- $header=$_POST['header'];
- $sidebar=$_POST['sidebar'];
- $footer=$_POST['footer'];
- $links=$_POST['links'];
- $text=$_POST['text'];
- mysql_query("UPDATE users SET background='$background',header='$header',sidebar='$sidebar',footer='$footer',texts='$text',links='$links' WHERE user_id='1'");
- $msg='Design updated.';
- }
- $sql=mysql_query("SELECT background,header,sidebar,footer,texts,links FROM users WHERE user_id='1'");
- $row=mysql_fetch_array($sql);
- $background=$row['background'];
- $header=$row['header'];
- $sidebar=$row['sidebar'];
- $footer=$row['footer'];
- $text=$row['texts'];
- $links=$row['links'];
- ?>
- <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
- <html xmlns="http://www.w3.org/1999/xhtml">
- <head>
- <meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
- <title>Jquery实时改变网页设计(背景色)</title>
- <meta content='Live Design Changing with Jquery' name='description'/>
- <meta content='Live, Design, Changing, with Jquery' name='keywords'/>
- <link rel="stylesheet" media="screen" type="text/css" href="css/colorpicker.css" />
- <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.3.0/jquery.min.js"></script>
- <script type="text/javascript" src="js/colorpicker.js"></script>
- <script type="text/javascript">
- $(document).ready(function()
- {
- $('.color').ColorPicker({
- onSubmit: function(hsb, hex, rgb, el) {
- $(el).val(hex);
- $(el).ColorPickerHide();
- },
- onBeforeShow: function () {
- $(this).ColorPickerSetColor(this.value);
- }
- })
- .bind('keyup', function(){
- $(this).ColorPickerSetColor(this.value);
- });
- $(".colorpicker_submit").click(function()
- {
- var B = $("#background").val();
- var sidebar = $("#sidebarinput").val();
- var header = $("#headerinput").val();
- var footer = $("#footerinput").val();
- var T = $("#textinput").val();
- var L = $("#linkinput").val();
- $("#header").css("background-color", "#"+header);
- $("#main_right").css("background-color", "#"+sidebar);
- $("#footer").css("background-color", "#"+footer);
- $('body').css("background-color", "#"+B);
- $('#container').css("color", "#"+T);
- $('#container a').css("color", "#"+L);
- });
- });
- </script>
- <style>
- *{ margin:0px; padding:0px}
- body
- {
- background-color:#<?php echo $background; ?>;
- font-family:Arial, Helvetica, sans-serif;
- }
- #container
- {
- margin:0 auto;
- width:800px;
- color:#<?php echo $text; ?>;
- }
- #container a
- {
- color:#<?php echo $links; ?>;
- }
- #header
- {
- background-color:#<?php echo $header; ?>;
- height:100px;
- margin-top:15px;
- }
- #main
- {
- min-height:600px;
- overflow:auto;
- margin-top:10px;
- }
- #main_left
- {
- background-color:#ffffff;
- min-height:600px;
- width:590px;
- margin-right:10px;
- overflow:auto;
- float:left;
- }
- #main_right
- {
- background-color:#<?php echo $sidebar; ?>;
- min-height:600px;
- width:200px;
- overflow:auto;
- float:left;
- }
- #footer
- {
- background-color:#<?php echo $footer; ?>;
- height:70px;
- margin-top:10px;
- }
- .rounded
- {
- border-radius:8px;
- -moz-border-radius:8px;
- -webkit-border-radius:8px;
- }
- .space
- {
- margin-bottom:15px;
- }
- label
- {
- font-size:13px;
- margin-bottom:5px;
- font-weight:bold;
- }
- .color
- {
- border:solid 1px #333;
- padding:4px;
- margin-top:8px;
- }
- .savebutton
- {
- color:#fff;
- border:solid 1px #333;
- padding:5px;
- background-color:#333;
- font-weight:bold
- }
- </style>
- </head>
- <body>
- <div id="container">
- <div id="header" class="rounded">
- </div>
- <div id="main">
- <div id="main_left" class="rounded">
- <div style="padding:25px">
- <h1>Live Design Changing with Jquery </h1>
- <br/>
- <form method="post" action="" />
- <div class="space">
- <label>Background:</label><br/> <input type="text" name="background" id="background" class="color" value="<?php echo $background; ?>" readonly="readonly"/>
- </div>
- <div class="space">
- <label>Header:</label> <br/><input type="text" name="header" class="color" id="headerinput" value="<?php echo $header; ?>" readonly="readonly" />
- </div>
- <div class="space">
- <label>Sidebar:</label><br/> <input type="text" name="sidebar" class="color" id="sidebarinput" value="<?php echo $sidebar; ?>" readonly="readonly"/>
- </div>
- <div class="space">
- <label>Footer:</label><br/> <input type="text" name="footer" class="color" id="footerinput" value="<?php echo $footer; ?>" readonly="readonly"/>
- </div>
- <div class="space">
- <label>Text:</label><br/> <input type="text" name="text" class="color" id="textinput" value="<?php echo $text; ?>" readonly="readonly"/>
- </div>
- <div class="space">
- <label>Links:</label><br/> <input type="text" name="links" class="color" id="linkinput" value="<?php echo $links; ?>" readonly="readonly"/>
- </div>
- <div class="space">
- <input type="submit" value=" Save Changes " class='savebutton'/> <?php echo $msg; ?>
- </div>
- </form>
- </div>
- </div>
- <div id="main_right" class="rounded">
- <div style="padding:10px"></div>
- </div>
- </div>
- <div id="footer" class="rounded">
- </div>
- </div>
- </div>
- </body>
- </html>
相关推荐
jQuery网页上下滚动背景变色特效,页面滚动改变背景颜色,背景颜色修改对应的data-background值即可。
网页设计 爱上jQuery 精巧实用的jquery插件
本文实例讲述了jquery实现实时改变网页字体大小、字体背景色和颜色的方法。分享给大家供大家参考。具体如下: 这里使用jquery实时改变字体大小、字体背景色和颜色,JQUERY让很多事变得更简单,确实是个实用的小插件...
jquery动态背景过渡是一款基于jQuery的网页背景特效,当你在表单输入内容切换到下一项时,背景颜色自动变幻,非常漂亮,创建动态背景的转换形式,一个简单而聪明的主意。专注于文本字段看到这种效果。
JavaScript+jQuery网页特效设计实例源码
Jquery实现的页面背景换色效果,可自己设置页面的背景颜色
jQuery点击按钮网页背景切换代码基于jquery.1.6.1.js制作,可切换5种不同网页背景。
JavaScript+jQuery网页特效设计任务驱动教程-PPT.zip
网页作业,使用HTML、CSS、JQuery、Bootstrap模仿网易云音乐网页界面
75款常用的jquery特效前端网页代码
jquery-gradientify是一款可以制作网页背景渐变色平滑过渡动画的jQuery插件。该插件使用简单,它通过参数的方式来提供一组渐变色,并使元素的背景色在这组渐变色之间反复平滑过渡。
全部的。 是《网页设计 爱上jQuery》书上的光盘所有的代码。
该部分内容是本作者基于Jquery和Bootstrap制作的课程设计,内容包括五个页面和相关的JS代码。
jQuery坦克大战网页小游戏.zip
jQuery点击弹窗设置网页背景颜色填充代码,鼠标点击右上角设置图标按钮弹出窗口输入16进制颜色值,应用后网页背景色即变成输入的颜色。
利用jquery根据照片自动计算出平均色作为照片背景,轻松实现最美照片背景色。
jQuery网页气泡动态背景特效是一款支持嵌套的jQuery网页动态背景特效代码。
jQuery网页视频背景插件是一款全屏网页mp4视频播放背景动画特效。
jQuery无刷新换肤,实时切换四种网页背景代码,适时切换四种网页背景颜色。选择网页右上角的四个彩色小按钮,单击一下,网页会将背景色变换为小按钮的颜色,并标记按钮。本实例为想实现无刷新换肤的朋友提供一种思路...